Plugs
Manage external hardware and resources with reusable logic.
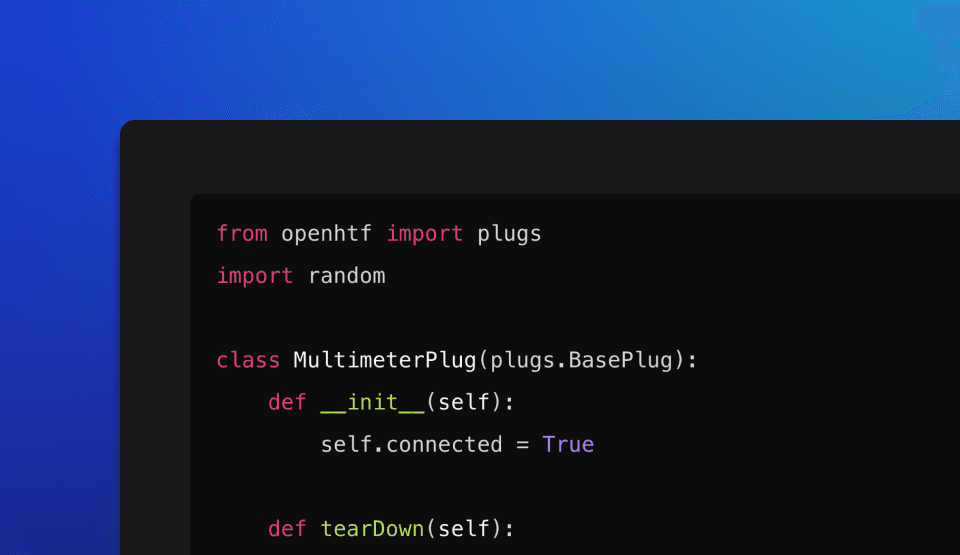
Overview
Hardware tests often require connections to external resources like instruments, UUTs, or other softwares. OpenHTF manages these connections through Plugs, reusable classes that handle resource initialization, control, and cleanup, separating test logic from resource management for easier maintenance and better logging.
Syntax
A plug is a standard Python class that inherits from BasePlug
.
multimeter_plug.py
from openhtf import plugs
import random
class MultimeterPlug(plugs.BasePlug):
def __init__(self):
# Simulate connecting to the multimeter
self.connected = True
def tearDown(self):
# Automatically called by OpenHTF after the test to clean up
self.connected = False
def measure_voltage(self):
# Simulate voltage measurement
return random.uniform(0, 10)
def measure_current(self):
# Simulate current measurement
return random.uniform(0, 2)
Usage
You can then use the plug
decorator in your test phases to inject the plug.
main.py
import openhtf as htf
from multimeter_plug import MultimeterPlug
@htf.plug(multimeter=MultimeterPlug)
def phase_test(test, multimeter):
# Use the plug to measure voltage and current
voltage = multimeter.measure_voltage()
current = multimeter.measure_current()
def main():
test = htf.Test(phase_test)
test.execute(lambda: "PCB001")
if __name__ == "__main__":
main()
OpenHTF manages the plug's lifecycle automatically:
-
Instantiation: At the start of the test, OpenHTF instantiates the plug by calling its
__init__()
method. -
Logging: During the test, the plug can log important actions or events using
self.logger
. -
Teardown: After the test concludes, OpenHTF automatically calls the
tearDown()
method to clean up resources. This ensures reliable cleanup since Python's destructors__del__()
aren't always called.
User Input Plug
You can use the UserInput
plug to prompt the operator during the test.
main.py
import openhtf as htf
from openhtf.plugs.user_input import UserInput
@htf.measures(
htf.Measurement("led_color")
.with_validator(lambda color: color in ["Red", "Green", "Blue"])
)
@htf.plug(user_input=UserInput)
def prompt_operator_led_color(test, user_input):
led_color = user_input.prompt(
message="What is the LED color? (Red/Green/Blue)"
)
test.measurements.led_color = led_color
def main():
test = htf.Test(prompt_operator_led_color)
test.execute(lambda: "PCB001")
if __name__ == "__main__":
main()
Terminal
What is the LED color? (Red/Green/Blue):
--> Red
======================= test: openhtf_test outcome: PASS ======================
Advanced use cases
You can use output callbacks extended features for advanced use cases.
Single plug configuration
You can define and load configurations specific to a plug, similar to test configurations.
multimeter_plug.py
from openhtf import BasePlug
from openhtf.util.configuration import CONF
# Define `com_port` configuration
CONF.declare("com_port", default_value="COM1")
class MultimeterPlug(BasePlug):
# Simulate connecting to the multimeter
def __init__(self):
self.com_port = CONF.com_port
self.connected = True
# Simulate measuring the voltage
def measure_voltage(self):
return 3.3
# Simulate disconnecting from the multimeter
# This method is called automatically by OpenHTF at the end of the test
def tearDown(self):
self.connected = False
Multiple plug configuration
You can use bind_init_args
to create multiple plug instances with variable configurations. This ensures flexible plug setup while maintaining compatibility with OpenHTF's automatic plug lifecycle management.
Example
from openhtf import BasePlug
class MultimeterPlug(BasePlug):
# Simulate connecting to the multimeter with port argument
def __init__(self, com_port: str):
self.com_port = com_port
self.connected = True
# Simulate measuring the voltage
def measure_voltage(self):
return 3.3
# Simulate disconnecting from the multimeter
# This method is called automatically by OpenHTF at the end of the test
def tearDown(self):
self.connected = False