Configurations
Control test execution parameters with configuration variables or files.
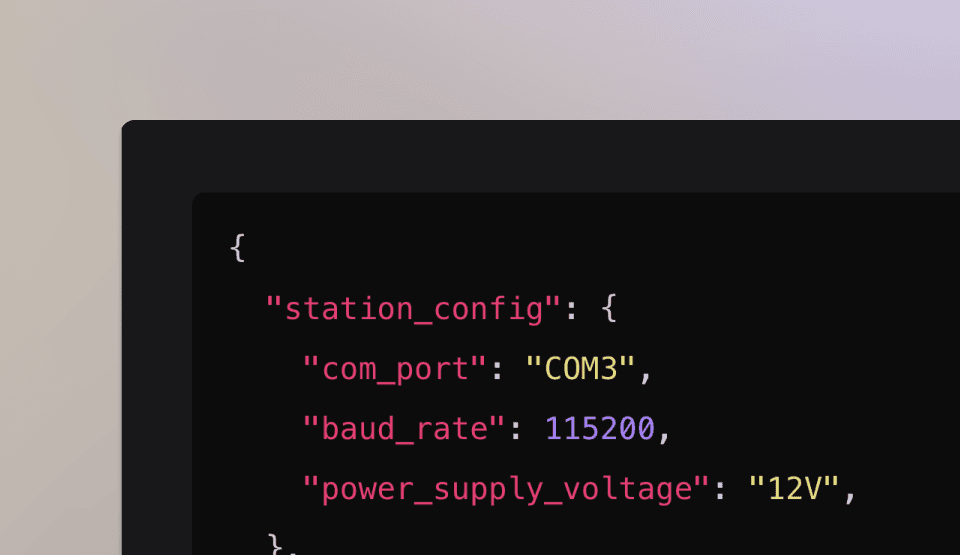
Overview
Sometimes you need to change script parameters without modifying the script itself, like updating firmware versions or adjusting instrument ports between stations. OpenHTF simplifies this by using configuration files to manage these parameters.
File
You can load configurations from an external file written in YAML or JSON format. For example:
config.yaml
firmware_path: 'path/to/firmware_v1.0.0.bin'
Load the configuration file during execution script:
main.py
import openhtf as htf
from openhtf.util import configuration
# Declare the firmware_path key
configuration.CONF.declare("firmware_path")
# Load configuration from an external YAML file
with open("config.yaml", "r") as yamlfile:
configuration.CONF.load_from_file(yamlfile)
def flash_firmware(test):
firmware_path = configuration.CONF.firmware_path
print(f"Flashing firmware from: {firmware_path}")
def main():
test = htf.Test(flash_firmware)
test.execute(test_start=lambda: "PCB001")
if __name__ == "__main__":
main()
Advanced use cases
Variables
You can set configuration variables directly in the script.
main.py
import openhtf as htf
from openhtf.util import configuration
# Define firmware_path configuration
configuration.CONF.declare(
"firmware_path",
default_value="path/to/firmware_v1.0.0.bin",
description="Path to the firmware file",
)
def flash_firmware(test):
firmware_path = configuration.CONF.firmware_path
print(f"Flashing firmware from: {firmware_path}")
def main():
test = htf.Test(flash_firmware)
test.execute(test_start=lambda: "PCB001")
if __name__ == "__main__":
main()
You can set the following parameters for each configuration variable in OpenHTF:
- Name
.name
- Type
- str
- Description
The unique identifier for the configuration variable (must be in snake_case).
- Name
.default_value
- Description
An optional value that serves as the default if no other value is provided.
- Name
.description
- Type
- str
- Description
An optional description explaining the purpose of the configuration variable.
Decorator
You can use the save_and_restore
decorator to temporarily change a value during the function call, which is then restored.
In this example, you can also configure the variable after its declaration.
main.py
import openhtf as htf
from openhtf.util import configuration
configuration.CONF.declare("phase_variance")
configuration.CONF.load(phase_variance=4)
@configuration.CONF.save_and_restore(phase_variance=55) # Decorator
def phase_one(test):
phase_variance_to_print = configuration.CONF.phase_variance
print(f"Value during phase one: {phase_variance_to_print}")
def phase_two(test):
phase_variance_to_print = configuration.CONF.phase_variance
print(f"Value during phase two: {phase_variance_to_print}")
def main():
test = htf.Test(phase_one, phase_two)
test.execute(test_start=lambda: "PCB001")
if __name__ == "__main__":
main()